Submitting a Form with Playwright - A Beginner’s Guide
Last updated 2 months ago
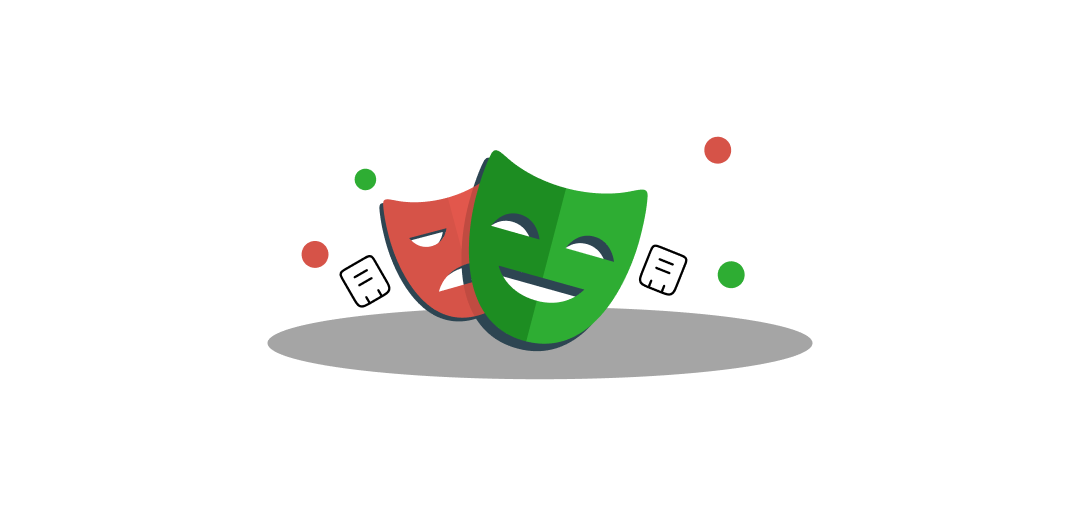
What is playwright? Playwright is a powerful end-to-end testing and automation library by Microsoft. It allows developers to write reliable tests and scripts for web applications across multiple browsers (Chromium, Firefox, and WebKit). One of the most common actions when automating web interactions is filling out and submitting a form. In this tutorial, we will walk through how to do exactly that with Playwright.
What You’ll Need
- Node.js installed on your machine (version 12 or higher).
- A basic understanding of JavaScript.
- Familiarity with the command line or terminal.
Setting Up Your Project
Create a new folder for your project. For instance:
mkdir playwright-form-example
cd playwright-form-example
Initialize your project with a package.json
file:
npm init -y
This automatically creates a basic package.json with default settings.
How To Install Playwright
npm install @playwright/test
By installing @playwright/test
, you get the Playwright test runner and the ability to launch browsers like Chromium, Firefox, and WebKit. Note: If you only need the core Playwright library, you can install playwright instead of @playwright/test
. But @playwright/test
is the recommended approach for testing scenarios.
Basic Project Structure
After installation, your folder might look like this:
playwright-form-example
│ package.json
│ package-lock.json
└── node_modules
Let’s create a file called form-submit.spec.js
(the .spec.js
extension is a convention for test files, but you can use .js
if you prefer).
Writing Your First Playwright Script
Below is a very simple Playwright example showing how to:
- Launch a browser (Chromium by default).
- Navigate to a sample website containing a form.
- Fill in the form fields (username and password).
- Click the submit button.
- Confirm the result.
// @ts-check
import { test, expect } from '@playwright/test';
test('submit a login form', async ({ page }) => {
// 1. Go to the page with the form
await page.goto('https://example-playwright-form.com/login');
// 2. Fill the form fields
await page.fill('#username', 'myTestUser');
await page.fill('#password', 'mySuperSecretPassword');
// 3. Click the submit button
await page.click('#submit-button');
// 4. Wait for the response page or success indicator
// (e.g., a welcome message)
const welcomeMessage = await page.waitForSelector('.welcome-text');
// 5. Check that the welcome message is visible
expect(await welcomeMessage.isVisible()).toBe(true);
// Optionally, check the text content
const messageText = await welcomeMessage.textContent();
expect(messageText).toContain('Welcome, myTestUser!');
});
Level Up Your Web Scraping Game!
Simply use the ScrapingBytes API. Get started for free with 1000 credits. No credit card required.
Sign Me Up!Explanation of the Code
Import statements:
import { test, expect } from '@playwright/test';
-
test
is a function from Playwright that allows you to define your test steps. -
expect
is used for assertions (verifying conditions like visibility or text content). -
test('submit a login form', async ({ page }) => { ... })
:- Defines a test named 'submit a login form'.
- Provides a
page
object which you can use to interact with the browser page.
-
await page.goto('https://example-playwright-form.com/login');
:- Navigates the browser to the page with the form you want to fill out.
Filling form fields:
await page.fill('#username', 'myTestUser');
await page.fill('#password', 'mySuperSecretPassword');
-
page.fill(selector, value)
selects an input element and types the specified value. - The CSS selectors here are
#username
and#password
, referencing theid
attribute in HTML.
Clicking the submit button:
await page.click('#submit-button');
- This simulates a user clicking on the button to submit the form.
Waiting for a response or success indicator:
const welcomeMessage = await page.waitForSelector('.welcome-text');
- Waits until an element with the class
.welcome-text
is available on the page. - This is handy because sometimes the page needs time to load or process the request after form submission.
Assertions (tests):
expect(await welcomeMessage.isVisible()).toBe(true);
const messageText = await welcomeMessage.textContent();
expect(messageText).toContain('Welcome, myTestUser!');
- We verify that the element is indeed visible (so the form submission was successful).
- We also check that the text content has the expected greeting.
Running the Test
To run the test- update your package.json
to add a script that runs Playwright tests:
{
"name": "playwright-form-example",
"version": "1.0.0",
"scripts": {
"test": "playwright test"
},
"devDependencies": {
"@playwright/test": "^1.0.0" // or your installed version
}
}
Run:
npm test
- Playwright will launch a headless browser (Chromium by default), run the test, and then provide a test report in your terminal.
Viewing the Test in a Non-Headless Browser
Sometimes, you’ll want to see the browser open and watch your script in action. To do so, run your tests with the --headed
flag:
npx playwright test --headed
This will open the browser so that you can observe the form submission flow.
Troubleshooting Tips
-
Selectors: Make sure your CSS selectors (like
#username
,#password
, and#submit-button
) match the actual HTML elements in the target page. -
Wait for Elements: If the page or certain elements take time to load, use Playwright’s waiting mechanisms, like
waitForSelector
or expect statements with.toBeVisible()
. - Environment Variables: For sensitive information (like real passwords), store them in environment variables, not hard-coded in your script.
Conclusion
Playwright makes it incredibly straightforward to automate web interactions, including filling and submitting forms. By using simple commands like page.fill()
and page.click()
, you can script an entire user flow. As a beginner, focus on understanding the basics of selectors, navigation, and waiting for elements. Once you grasp these concepts, you’ll be well on your way to writing more complex tests and scripts like Playwright API testing, authentication flows, and multi-page navigation.